Failure to correctly nest functions may result in Data Blocks not functioning properly and/or Data Blocks not being exported/imported as expected via the Assette VS Code PLugin.
When creating a Data Block, developers may sometimes want to create nested functions (also sometimes called “Inner Functions” or “Sub-functions”) to help organize their code. Assette Data Blocks do allow developers to use nested functions, however, it is important to note that any functions declared within another function (i.e., a nested function) must either be defined globally or called separately within a Data Block. Shown below, sub_function1 is not included in the scope sub_function2(), and therefore is sub_function1() is defined globally at the beginning of the script.
#----Code start identification-do not remove this line---
global sub_function1
def sub_function1(func_params1):
# Sub-function 1 goes here
return func_params1*2
def sub_function2(func_params2):
# Sub-function 1 goes here
return sub_function1(func_params2)*func_params2
final_result = dict()
# Call the sub-functions and do activities to populate the final_result
final_result["answer"] = sub_function2(params["num1"])
response["data"] = final_result
#----Code end identification-do not remove this line---
If one were to preview the results via the Data Block Editor, they would see that the value of “answer” is 18. However, attempting to run the above code snippet of code directly in VS Code produces a NameError, as response has not been defined.
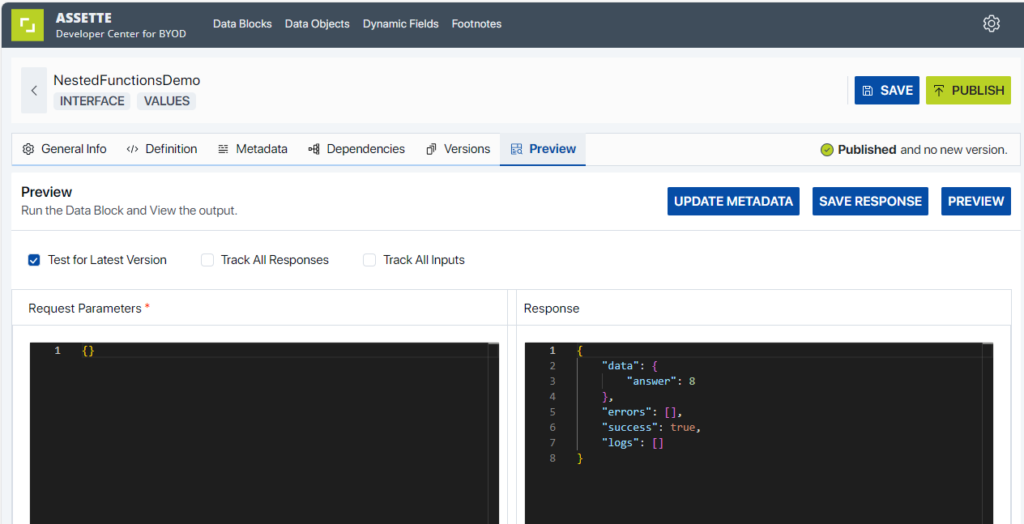
To run in an IDE, simply wrap the Data Block’s code, leaving “global” in place, as shown below.
def execute(response, read, params):
# Main activity goes here
#----Code start identification-do not remove this line---
global sub_function1
def sub_function1(func_params1):
# Sub-function 1 goes here
return func_params1*2
def sub_function2(func_params2):
# Sub-function 1 goes here
return sub_function1(func_params2)*func_params2
final_result = dict()
# Call the sub-functions and do activities to populate the final_result
final_result["answer"] = sub_function2(params["num1"])
response["data"] = final_result
#----Code end identification-do not remove this line---
if __name__ == "__main__":
response = {}
execute(response, None, {"num1":3})
print(response)
Then when importing via Assette’s VS Code Plugin, only the inner block, including the global definition, is imported to Assette and the Data Block can be run ‘as is’.
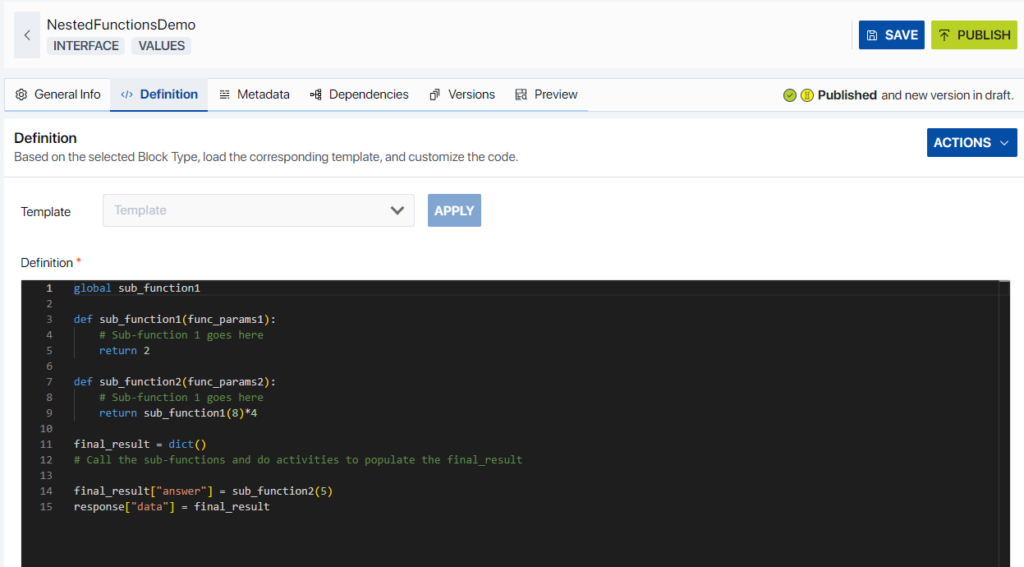